A Template function to return minimum of 2 values.
Here is a very minimal example of a templete.
You should make sure that you understand this, before going on to more examples.
// Programmer: Janet Joy
// Example of template
#include <iostream>
#include<string>
using namespace std;
template <typename T>
T minimum( T val1, T val2)
{
// Because it is template, it can accept any type that has < .
T result = val1;
if (val2 < val1) result = val2;
return result;
}
int main() {
int num=5;
// Test with int
cout << minimum(num, 3)<<endl;
// Test with double
double d1 = 3.5, d2 = 7.1;
cout << minimum(d1, d2) << endl;
// Test with character
char c1 = 'a', c2 = 'b';
cout << minimum(c1, c2) << endl;
string s1 = "hello", s2 = "goodbye";
cout << minimum(s1, s2) << endl;
system("pause");
return 0;
}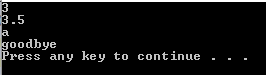