Order the List
The two buttons allow use to move the selected item in the list up and down.
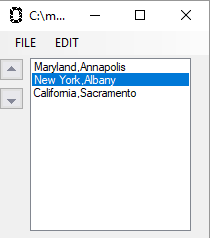
Write the code as shown below.
private void btnUp_Click(object sender, EventArgs e)
{
// Change the position by -1 example: Move from position 3 to position 2
MoveItem(-1);
}
private void btnDown_Click(object sender, EventArgs e)
{
// Change the position by 1 example: Move from position 2 to position 3
MoveItem(1);
}
public void MoveItem(int direction)
{
// Check if item can it be moved.
Boolean ok = true;
// Checking selected item
if (lstData.SelectedItem == null || lstData.SelectedIndex < 0)
ok = false; // No selected item - nothing to do
// Calculate new index using move direction
int newIndex = lstData.SelectedIndex + direction;
// Checking bounds of the range
if (newIndex < 0 || newIndex >= lstData.Items.Count)
ok = false; // Index out of range - nothing to do
if (ok)
{ // None of the errors above occured, move the item.
object selected = lstData.SelectedItem;
// Removing removable element
lstData.Items.Remove(selected);
// Insert it in new position
lstData.Items.Insert(newIndex, selected);
// Restore selection
lstData.SetSelected(newIndex, true);
changed = true;
}
}
To Do:Make sure everything works!
Experiment:When the form is resized, make hte list box fill the area.
Complete code for listbox editor