Start a new project named Cryptogram. Build the form as shown below:
- Add a label
with the words "Enter a saying, then press the button to make a cryptogram"
- Add a text box
named TxtWords.
- Set multiline to true for the text box.
- Copy TxtWords and paste. Rename as TxtCryptogram.
- Add a button
. Name it BtnGo and make the text &Go
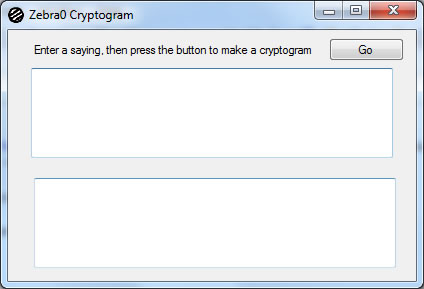
Write the code as shown below:
Public Class Form1
Private Sub BtnGo_Click(sender As Object, e As EventArgs) Handles BtnGo.Click
Dim Alphabet As String = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
Dim Code As String = Scramble(Alphabet)
Me.Text = Code
Dim S As String = Me.TxtWords.Text
S = S.ToUpper
Dim S2 As String = ""
Dim I, Pos As Integer
For I = 0 To S.Length - 1
Dim Ch As Char = S.Substring(I, 1) 'get 1 character from S
Pos = Alphabet.IndexOf(Ch)
If Pos >= 0 Then
S2 += Code.Substring(I, 1) 'get the corresponding character from code
Else
S2 += Ch 'if not a letter, do not encode it
End If
Next
Me.TxtCryptogram.Text = S2
End Sub
Private Function Scramble(ByVal S As String) As String
Dim Ch As Char()
Ch = S.ToCharArray()
Dim I As Integer
For I = 0 To Ch.Length - 1
Dim R As Integer = Int(Rnd() * Ch.Length)
Dim Temp As Char = Ch(I)
Ch(I) = Ch(R)
Ch(R) = Temp
Next
Dim S2 As String = ""
For I = 0 To Ch.Length - 1
S2 += Ch(I)
Next
Return S2
End Function 'Scramble
Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
Randomize() 'This make sure it is different each time you run it
End Sub
End Class
Experiment! You can make lots of games like hangman, fortune, etc. that use strings.