A regular expression is a way to determine if a string matches a pattern.
The regular expression syntax can be rather complex.
We will start with a fairly simple example: whether a string is a valid Social Security number.
Start a new project named Regular. Build the form as shown below
: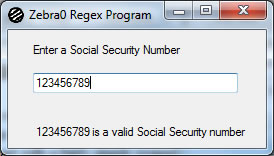
- There is a label
with the words "Enter a Social Security Number and press Enter"
- There is a text box
named TxtSoc.
- There is a label
named LblMessage
For now we will assume that the only valid string is 9 digits. We can represent that pattern as [0-9]{9} meaning that an element in the set of digits [0-9] must be repeated {9} times.
Write the code as shown below. Notice that you must import System.Text.RegularExpressions
Imports System.Text.RegularExpressions
Public Class Form1
Private Sub TxtSoc_KeyPress(sender As Object, e As KeyPressEventArgs) Handles TxtSoc.KeyPress
If e.KeyChar.Equals(Chr(13)) Then 'If they pressed Enter
Dim Soc As String = TxtSoc.Text
Dim SocRegex As Regex = New Regex("[0-9]{9}")
Dim M As Match = SocRegex.Match(Soc)
If M.ToString = Soc Then 'They should be the same if it was accepted
LblMessage.Text = Soc & " is a valid Social Security number"
Else
LblMessage.Text = Soc & " is NOT a valid Social Security number"
End If 'check if input matches regex expression
End If 'pressed enter
End Sub 'Key press
End Class 'Form